Central themes: Starting an App, getting Kivy running
It’s compulsory that the introduction to any programming project should contain a "Hello World!" application. Since Kivy is a GUI framework, that means starting an application and displaying the words on the screen. Start by adding each of the following lines to your program:
from kivy.app import App
This imports the App class, something you’ll be using in any Kivy application. Your instance of this class will create the Kivy window and serve as the top level of your application
from kivy.uix.label import Label
This introduces one of Kivy’s most important components; the Widget. Your entire application will be built with Widgets, each of which does a single (relatively) small task. For instance, Label is a Widget that displays some text, Button is (obviously) a button, and Layouts are Widgets that contain other Widgets and lay them out in some particular arrangement.
You can find the documentation for Label here. We’ll need this later!.
In every Kivy application, your first task is to create an App subclass as follows:
class YourApp(App):
def build(self):
root_widget = Label(text='Hello world!')
return root_widget
The build method is the only important addition you have to make, and is the usually the first entry point for your use of a Kivy application. This method must instantiate and return what will be your root widget, the top level widget of your Kivy application, which will contain anything else you add.
The root widget will automatically fill the window, so in this case the Label text will appear right in the middle.
In our case, the application will only ever need a single Widget; the Label displaying our text. We set the text by simply passing it as an argument. This works automatically because text is a Kivy property of the Label widget…but that doesn’t matter right now.
Finally, add a line to start and run the Application:
YourApp().run()
This instantiates and runs the instance of your App. Any Kivy application is created and started with some variation of these basic steps.
Now…run the code!
python your_filename.py
You should see a Window something like the following image. Congratulations, you’ve written and run your first Kivy application.
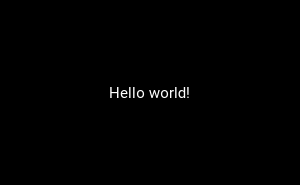
Full code
your_filename.py:
from kivy.app import App
from kivy.uix.label import Label
class YourApp(App):
def build(self):
root_widget = Label(text='Hello world!')
return root_widget
YourApp().run()